Note
Click here to download the full example code
VAMP on Position Based Fluids¶
Projection of position based fluids simulation timeseries on dominant component. For more details, see the VAMP tutorial.
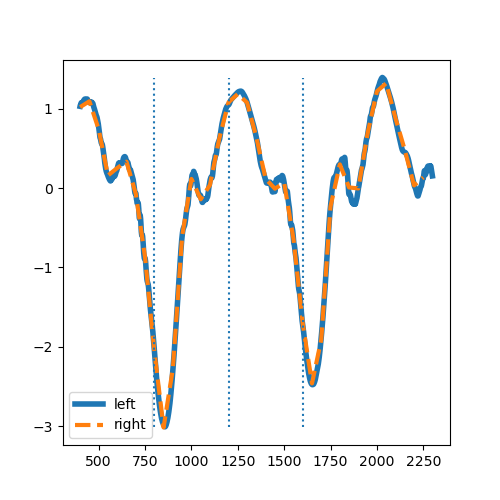
9 import matplotlib.pyplot as plt
10 import numpy as np
11
12 from deeptime.data import position_based_fluids
13 from deeptime.decomposition import VAMP
14
15 pbf_simulator = position_based_fluids(n_burn_in=500, n_jobs=8)
16 trajectory = pbf_simulator.simulate_oscillatory_force(n_oscillations=3, n_steps=400)
17 n_grid_x = 20
18 n_grid_y = 10
19 kde_trajectory = pbf_simulator.transform_to_density(
20 trajectory, n_grid_x=n_grid_x, n_grid_y=n_grid_y, n_jobs=8
21 )
22 tau = 100
23 model = VAMP(lagtime=100).fit(kde_trajectory).fetch_model()
24 projection_left = model.forward(kde_trajectory, propagate=False)
25 projection_right = model.backward(kde_trajectory, propagate=False)
26
27 f, ax = plt.subplots(1, 1, figsize=(5, 5))
28 start = 400
29 stop = len(kde_trajectory) - tau # 5000
30 left = projection_left[:-tau][start:stop, 0]
31 right = projection_right[tau:][start:stop, 0]
32 lw = 4
33 ax.plot(np.arange(start, stop), left, label="left", linewidth=lw)
34 ax.plot(np.arange(start, stop)[::50], right[::50], '--', label="right", linewidth=3, markersize=12)
35 ax.vlines([start + i * 400 for i in range(1, (stop - start) // 400)], np.min(left), np.max(left),
36 linestyles='dotted')
37 ax.legend()
Total running time of the script: ( 0 minutes 12.661 seconds)
Estimated memory usage: 45 MB