Note
Click here to download the full example code
Coherent sets: Kernel CCA on the Bickley jet¶
This example shows an application of KernelCCA
on the
bickley jet
dataset. One can cluster in the singular function space
to find coherent structures.
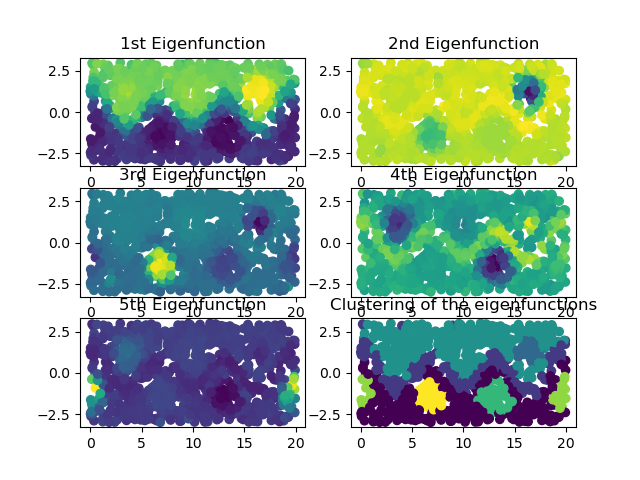
9 import matplotlib.pyplot as plt
10 import numpy as np
11
12 from deeptime.clustering import KMeans
13 from deeptime.data import bickley_jet
14 from deeptime.decomposition import KernelCCA
15 from deeptime.kernels import GaussianKernel
16
17 dataset = bickley_jet(n_particles=1000, n_jobs=8).endpoints_dataset()
18 kernel = GaussianKernel(.7)
19
20 estimator = KernelCCA(kernel, n_eigs=5, epsilon=1e-3)
21 model = estimator.fit((dataset.data, dataset.data_lagged)).fetch_model()
22
23 ev_real = np.real(model.eigenvectors)
24 kmeans = KMeans(n_clusters=7, n_jobs=8).fit(ev_real)
25 kmeans = kmeans.fetch_model()
26
27 fig = plt.figure()
28 gs = fig.add_gridspec(ncols=2, nrows=3)
29
30 ax = fig.add_subplot(gs[0, 0])
31 ax.scatter(*dataset.data.T, c=ev_real[:, 0])
32 ax.set_title('1st Eigenfunction')
33
34 ax = fig.add_subplot(gs[0, 1])
35 ax.scatter(*dataset.data.T, c=ev_real[:, 1])
36 ax.set_title('2nd Eigenfunction')
37
38 ax = fig.add_subplot(gs[1, 0])
39 ax.scatter(*dataset.data.T, c=ev_real[:, 2])
40 ax.set_title('3rd Eigenfunction')
41
42 ax = fig.add_subplot(gs[1, 1])
43 ax.scatter(*dataset.data.T, c=ev_real[:, 3])
44 ax.set_title('4th Eigenfunction')
45
46 ax = fig.add_subplot(gs[2, 0])
47 ax.scatter(*dataset.data.T, c=ev_real[:, 4])
48 ax.set_title('5th Eigenfunction')
49
50 ax = fig.add_subplot(gs[2, 1])
51 ax.scatter(*dataset.data.T, c=kmeans.transform(ev_real))
52 ax.set_title('Clustering of the eigenfunctions')
Total running time of the script: ( 0 minutes 4.222 seconds)
Estimated memory usage: 106 MB