Note
Click here to download the full example code
2D densities from xy¶
This example demonstrates how to plot unordered xy data - in this case, particle positions (xy) - as contour of their
density in both linear and log scale. See deeptime.plots.plot_density()
.
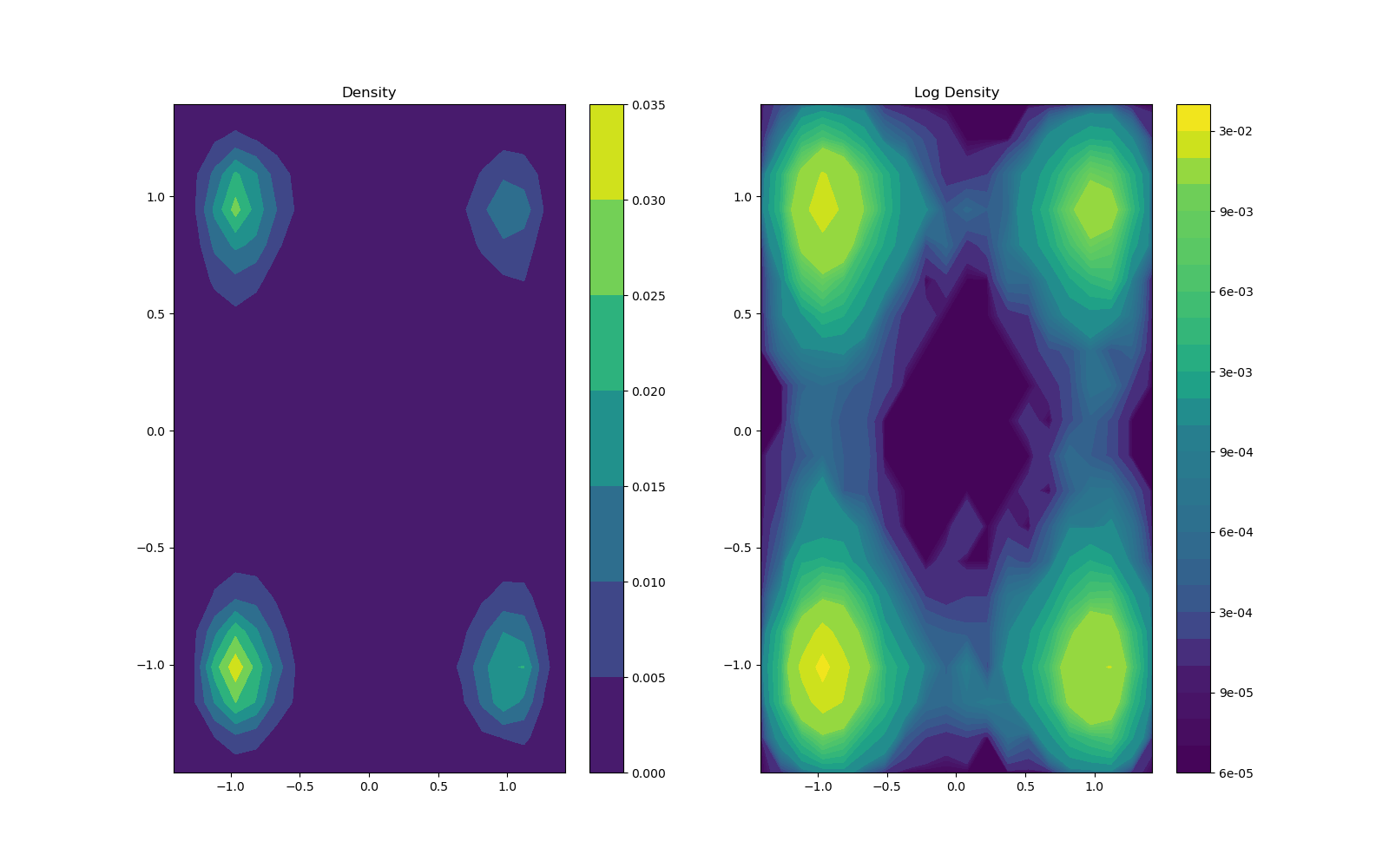
9 import numpy as np
10
11 import matplotlib.pyplot as plt
12 from matplotlib import ticker
13
14 from deeptime.data import quadruple_well
15 from deeptime.plots import plot_density
16
17 system = quadruple_well(h=1e-3, n_steps=100)
18 trajs = system.trajectory(x0=[[-1, 0], [1, 0], [0, 0]], length=5000)
19 traj_concat = np.concatenate(trajs, axis=0)
20
21 f, (ax1, ax2) = plt.subplots(1, 2, figsize=(16, 10))
22
23 ax1.set_title('Density')
24 ax1, mappable1 = plot_density(*traj_concat.T, n_bins=20, contourf_kws=dict(), ax=ax1)
25 f.colorbar(mappable1, ax=ax1, format=ticker.FuncFormatter(lambda x, pos: f"{x:.3f}"))
26
27 ax2.set_title('Log Density')
28 contourf_kws = dict(locator=ticker.LogLocator(base=10, subs=range(1, 10)))
29 ax2, mappable2 = plot_density(*traj_concat.T, n_bins=20, avoid_zero_counts=True, contourf_kws=contourf_kws, ax=ax2)
30 f.colorbar(mappable2, ax=ax2, format=ticker.FuncFormatter(lambda x, pos: f"{x:.0e}"))
Total running time of the script: ( 0 minutes 0.655 seconds)
Estimated memory usage: 9 MB