Note
Click here to download the full example code
Quadruple-well¶
Example for the deeptime.data.quadruple_well()
dataset.
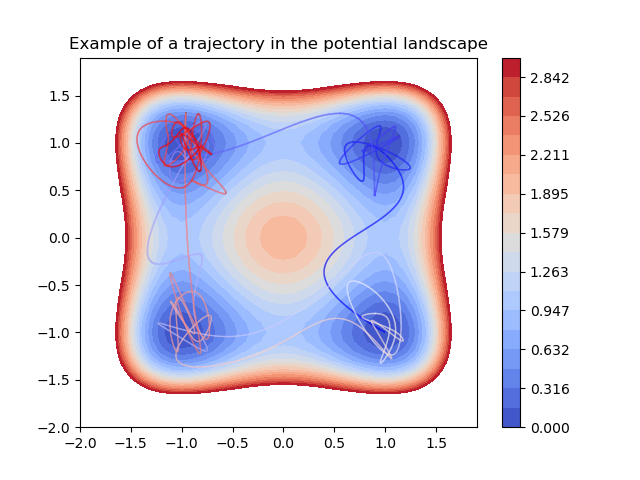
8 import numpy as np
9 import scipy
10 import matplotlib.pyplot as plt
11 from matplotlib.collections import LineCollection
12
13 from deeptime.data import quadruple_well
14
15 system = quadruple_well(n_steps=1000)
16 traj = system.trajectory(np.array([[1, -1]]), 100, seed=46)
17
18 xy = np.arange(-2, 2, 0.1)
19 coords = np.dstack(np.meshgrid(xy, xy)).reshape(-1, 2)
20 V = system.potential(coords).reshape((xy.shape[0], xy.shape[0]))
21
22 fig, ax = plt.subplots(1, 1)
23 ax.set_title("Example of a trajectory in the potential landscape")
24
25 cb = ax.contourf(xy, xy, V, levels=np.linspace(0.0, 3.0, 20), cmap='coolwarm')
26
27 x = np.r_[traj[:, 0]]
28 y = np.r_[traj[:, 1]]
29 f, u = scipy.interpolate.splprep([x, y], s=0, per=False)
30 xint, yint = scipy.interpolate.splev(np.linspace(0, 1, 50000), f)
31
32 points = np.stack([xint, yint]).T.reshape(-1, 1, 2)
33 segments = np.concatenate([points[:-1], points[1:]], axis=1)
34 coll = LineCollection(segments, cmap='bwr')
35 coll.set_array(np.linspace(0, 1, num=len(points), endpoint=True))
36 coll.set_linewidth(1)
37 ax.add_collection(coll)
38
39 fig.colorbar(cb)
Total running time of the script: ( 0 minutes 0.698 seconds)
Estimated memory usage: 28 MB