Note
Click here to download the full example code
Sqrt model¶
Sample a hidden state and an sqrt-transformed emission trajectory. Demonstrates deeptime.data.sqrt_model()
.
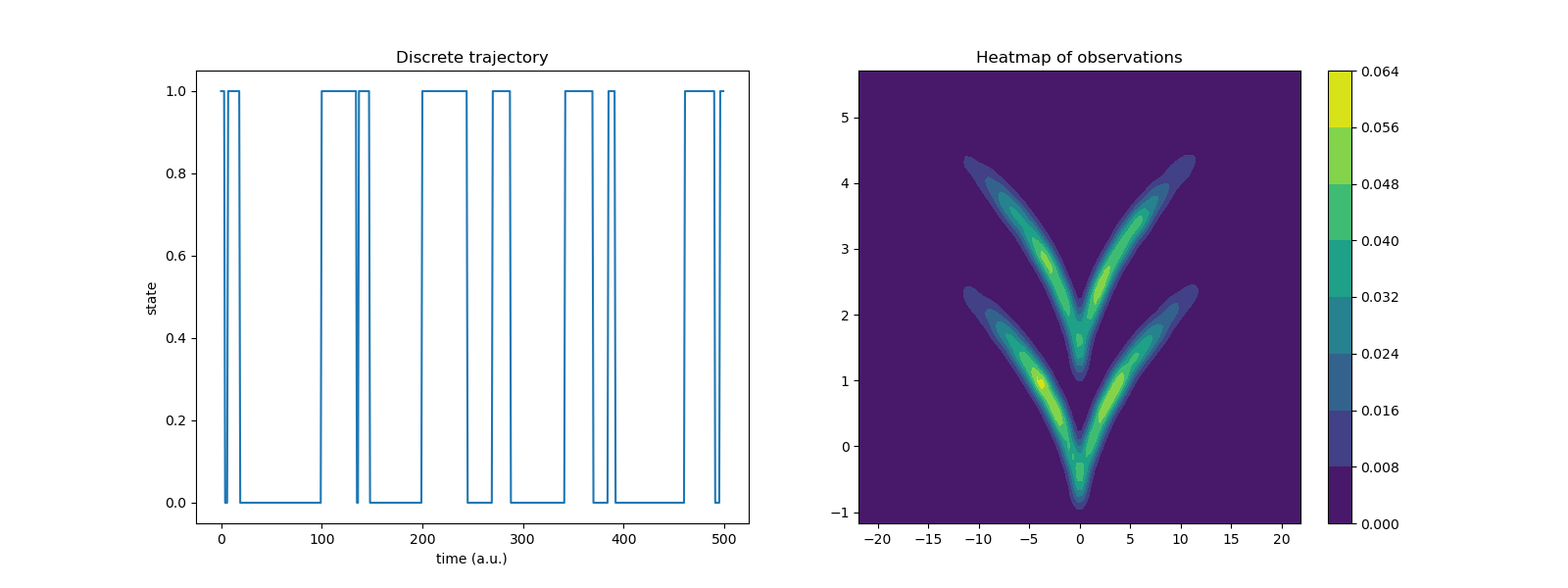
8 import numpy as np
9 import matplotlib.pyplot as plt
10 import scipy.stats as stats
11
12 from deeptime.data import sqrt_model
13
14 n_samples = 10000
15 dtraj, traj = sqrt_model(n_samples)
16
17 X, Y = np.meshgrid(
18 np.linspace(np.min(traj[:, 0]), np.max(traj[:, 0]), 100),
19 np.linspace(np.min(traj[:, 1]), np.max(traj[:, 1]), 100),
20 )
21 kde_input = np.dstack((X, Y)).reshape(-1, 2)
22
23 kernel = stats.gaussian_kde(traj.T, bw_method=.1)
24 Z = kernel(kde_input.T).reshape(X.shape)
25
26 f, (ax1, ax2) = plt.subplots(1, 2, figsize=(16, 6))
27 ax1.plot(dtraj[:500])
28 ax1.set_title('Discrete trajectory')
29 ax1.set_xlabel('time (a.u.)')
30 ax1.set_ylabel('state')
31
32 cm = ax2.contourf(X, Y, Z)
33 plt.colorbar(cm, ax=ax2)
34 ax2.set_title('Heatmap of observations')
Total running time of the script: ( 0 minutes 2.127 seconds)
Estimated memory usage: 9 MB