Note
Click here to download the full example code
Thomas attractor¶
The Thomas attractor. See deeptime.data.thomas_attractor()
.
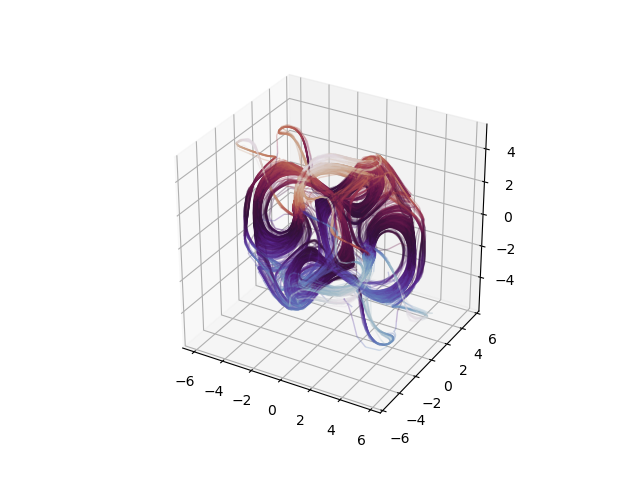
8 import deeptime
9 import numpy as np
10 import matplotlib.pyplot as plt
11 from mpl_toolkits.mplot3d.art3d import Line3DCollection
12
13 system = deeptime.data.thomas_attractor(.1)
14
15 x0 = np.random.uniform(-3, 3, size=(50, 3))
16 traj = system.trajectory(x0, 500)
17 tstart = 0
18 tfinish = system.h * system.n_steps * len(traj)
19
20 ax = plt.figure().add_subplot(projection='3d')
21
22 for t in traj:
23 points = t.reshape((-1, 1, 3))
24 segments = np.concatenate([points[:-1], points[1:]], axis=1)
25 coll = Line3DCollection(segments, cmap='twilight', alpha=.3)
26 coll.set_array(t[:, -1])
27 coll.set_linewidth(1)
28 ax.add_collection(coll)
29
30 ax.plot(*traj[0].T, alpha=0)
31 ax.set_box_aspect(np.ptp(traj[0], axis=0))
Total running time of the script: ( 0 minutes 1.868 seconds)
Estimated memory usage: 8 MB